import sensor, image, time
sensor.reset() # Initialize the camera sensor.
sensor.set_pixformat(sensor.RGB565) # use RGB565.
sensor.set_framesize(sensor.QQVGA) # use QVGA for quailtiy ,use QQVGA for speed.
sensor.skip_frames(10) # Let new settings take affect.
sensor.set_auto_whitebal(False)
#关闭白平衡。白平衡是默认开启的,在颜色识别中,需要关闭白平衡。
clock = time.clock() # Tracks FPS.
weed_threshold_01 = ((31, 100, -128, -12, 0, 127))
'''
扩宽roi
'''
def expand_roi(roi):
# set for QQVGA 160*120
extra = 5
win_size = (160, 120)
(x, y, width, height) = roi
new_roi = [x-extra, y-extra, width+2*extra, height+2*extra]
if new_roi[0] < 0:
new_roi[0] = 0
if new_roi[1] < 0:
new_roi[1] = 0
if new_roi[2] > win_size[0]:
new_roi[2] = win_size[0]
if new_roi[3] > win_size[1]:
new_roi[3] = win_size[1]
return tuple(new_roi)
while(True):
clock.tick()
img = sensor.snapshot()
blobs = img.find_blobs([weed_threshold_01], area_threshold=150)
if blobs:
#如果找到了目标颜色
# print(blobs)
for blob in blobs:
#迭代找到的目标颜色区域
is_rect = False
max_area = 0
new_roi = expand_roi(blob.rect())
rects=img.find_rects(roi=new_roi,threshold = 10000)
if rects:
is_rect=True
for rect in rects:
print(rects)
if rect.w()*rect.h() > max_area:
max_area = rect.w()*rect.h()
max_circle = rect
img.draw_rectangle(max_circle.x(),max_circle.y(),max_circle.w(),max_circle.h())
print(clock.fps())
2ecb
@2ecb
2ecb 发布的帖子
-
MemoryError:Out of fast Frame Buffer Stack Memory!
-
求问LAB这三个通道的数值对于识别色块各有什么影响?
求问LAB这三个通道的数值对于识别色块各有什么影响?
比如说对于识别一个色块,阈值调好了,然后如果稍微改变L的值,对其有什么影响吗? -
保存特征点出现OSError:Failed to write requested bytes!
Keypoints descriptor example.
This example shows how to save a keypoints descriptor to file. Show the camera an object
and then run the script. The script will extract and save a keypoints descriptor and the image.
You can use the keypoints_editor.py util to remove unwanted keypoints.
NOTE: Please reset the camera after running this script to see the new file.
import sensor, time, image
Reset sensor
sensor.reset()
Sensor settings
sensor.set_contrast(3)
sensor.set_gainceiling(16)
sensor.set_framesize(sensor.VGA)
sensor.set_windowing((320, 240))
sensor.set_pixformat(sensor.GRAYSCALE)sensor.skip_frames(time = 2000)
sensor.set_auto_gain(False, value=100)FILE_NAME = "alphabet_A2"
img = sensor.snapshot()NOTE: See the docs for other arguments
NOTE: By default find_keypoints returns multi-scale keypoints extracted from an image pyramid.
A= img.find_keypoints(max_keypoints=150, threshold=10, scale_factor=1.2)
if (A == None):
raise(Exception("Couldn't find any keypoints!"))image.save_descriptor(A, "/%s.orb"%(FILE_NAME))
img.save("/%s.pgm"%(FILE_NAME))img.draw_keypoints(A)
sensor.snapshot()
time.sleep(1000)
raise(Exception("Done! Please reset the camera")) -
RE: AttributeError:'Inage' object has no attribute 'find_number'
image对象中没有find_number吗?
-
AttributeError:'Inage' object has no attribute 'find_number'
# LetNet Example import sensor, image, time sensor.reset() # Reset and initialize the sensor. sensor.set_contrast(3) sensor.set_pixformat(sensor.GRAYSCALE) # Set pixel format to RGB565 (or GRAYSCALE) sensor.set_framesize(sensor.QVGA) # Set frame size to QVGA (320x240) sensor.set_windowing((28, 28)) sensor.skip_frames(time = 2000) # Wait for settings take effect. sensor.set_auto_gain(False) sensor.set_auto_exposure(False) clock = time.clock() # Create a clock object to track the FPS. while(True): clock.tick() # Update the FPS clock. img = sensor.snapshot() # Take a picture and return the image. out = img.invert().find_number() if out[1] > 3.0: print(out[0]) #print(clock.fps()) # Note: OpenMV Cam runs about half as fast when connected # to the IDE. The FPS should increase once disconnected.
-
出现报错 OSError: [Errno 19] ENODEV 实例30:distance_read
import pyb
VL51L1X_DEFAULT_CONFIGURATION = bytes([
0x00,
0x00,
0x00,
0x01,
0x02,
0x00,
0x02,
0x08,
0x00,
0x08,
0x10,
0x01,
0x01,
0x00,
0x00,
0x00,
0x00,
0xff,
0x00,
0x0F,
0x00,
0x00,
0x00,
0x00,
0x00,
0x20,
0x0b,
0x00,
0x00,
0x02,
0x0a,
0x21,
0x00,
0x00,
0x05,
0x00,
0x00,
0x00,
0x00,
0xc8,
0x00,
0x00,
0x38,
0xff,
0x01,
0x00,
0x08,
0x00,
0x00,
0x01,
0xdb,
0x0f,
0x01,
0xf1,
0x0d,
0x01,
0x68,
0x00,
0x80,
0x08,
0xb8,
0x00,
0x00,
0x00,
0x00,
0x0f,
0x89,
0x00,
0x00,
0x00,
0x00,
0x00,
0x00,
0x00,
0x01,
0x0f,
0x0d,
0x0e,
0x0e,
0x00,
0x00,
0x02,
0xc7,
0xff,
0x9B,
0x00,
0x00,
0x00,
0x01,
0x01,
0x40
])
class VL53L1X:
def init(self,i2c, address=0x29):
self.i2c = i2c
self.address = address
self.reset()
pyb.delay(1)
if self.read_model_id() != 0xEACC:
raise RuntimeError('Failed to find expected ID register values. Check wiring!')
self.i2c.writeto_mem(self.address, 0x2D, VL51L1X_DEFAULT_CONFIGURATION, addrsize=16)
self.writeReg16Bit(0x001E, self.readReg16Bit(0x0022) * 4)
pyb.delay(200)
def writeReg(self, reg, value):
return self.i2c.writeto_mem(self.address, reg, bytes([value]), addrsize=16)
def writeReg16Bit(self, reg, value):
return self.i2c.writeto_mem(self.address, reg, bytes([(value >>& 0xFF, value & 0xFF]), addrsize=16)
def readReg(self, reg):
return self.i2c.readfrom_mem(self.address, reg, 1, addrsize=16)[0]
def readReg16Bit(self, reg):
data = self.i2c.readfrom_mem(self.address, reg, 2, addrsize=16)
return (data[0]<<8) + data[1]
def read_model_id(self):
return self.readReg16Bit(0x010F)
def reset(self):
self.writeReg(0x0000, 0x00)
pyb.delay(100)
self.writeReg(0x0000, 0x01)
def read(self):
data = self.i2c.readfrom_mem(self.address, 0x0089, 17, addrsize=16)
range_status = data[0]
stream_count = data[2]
dss_actual_effective_spads_sd0 = (data[3]<<8) + data[4]
ambient_count_rate_mcps_sd0 = (data[7]<<8) + data[8]
final_crosstalk_corrected_range_mm_sd0 = (data[13]<<8) + data[14]
peak_signal_count_rate_crosstalk_corrected_mcps_sd0 = (data[15]<<8) + data[16]
return final_crosstalk_corrected_range_mm_sd0 -
OPENMV定时器发送数据报错
import sensor, image, time from pyb import UART from pyb import millis from math import pi, isnan import time from pyb import Pin, Timer #from pid import PID #若优化在cricket_test.py修改 cricket_control.py为最终函数 def tick(timer): #print("Timer callback") output_str="[%d,%d]" % (x_now,y_now) uart.write(output_str+'\r\n') print(max_blob.cx(),max_blob.cy()) #2个 tim = Timer(4, freq=1) tim.callback(tick) sensor.reset() sensor.set_framesize(sensor.QVGA) sensor.set_pixformat(sensor.RGB565) sensor.skip_frames(time = 2000) clock = time.clock() uart = UART(3, 115200) red_threshold = ((34, 63, 27, 80, 3, 127)) x_now=y_now=0 def find_max(blobs): max_size=0 for blob in blobs: if blob[2]*blob[3] > max_size: max_blob=blob max_size = blob[2]*blob[3] return max_blob while(True): clock.tick() img = sensor.snapshot() blobs = img.find_blobs([red_threshold],roi=(55,14,225,218),pixels_threshold=2, area_threshold=2) if blobs: max_blob = find_max(blobs) img.draw_rectangle(max_blob.rect()) img.draw_cross(max_blob.cx(), max_blob.cy(),color=0) x_now=max_blob.cx() #x_now:x方向上现在的色块中心坐标位置 y_now=max_blob.cy() #y_now:y方向上现在的色块中心坐标位置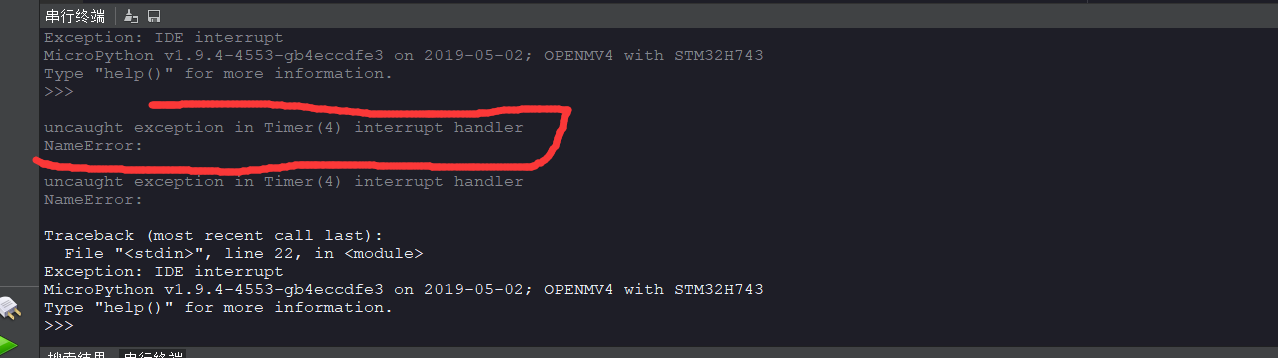
-
RE: 想用定时器打印找到的色块的中心坐标,为什么程序会卡死,出现忙碌中....求救!!!
@kidswong999 好的!本来是想固定时间进行PID的,那您说的是利用millis()在死循环刷吗?(到达一定时间后再进行判断操作执行PID)